If your terminal does not support ANSI console features, this class will fall back to safer, more standard output, rather than cluttering the screen with jibberish. As such, this library is a great tool for creating stylish console apps without having to worry about which terminal features may be available for all end users.
- Loading the Console Output Library
- Default Styles
- Supported methods
- Constructor
- ConsoleOutput:setStyle()
- ConsoleOutput:isAnsiAvailable()
- ConsoleOutput:write()
- ConsoleOutput:writeln()
- ConsoleOutput:sstyle()
- ConsoleOutput:style()
- ConsoleOutput:default()
- ConsoleOutput:petty()
- ConsoleOutput:info()
- ConsoleOutput:comment()
- ConsoleOutput:success()
- ConsoleOutput:fail()
- ConsoleOutput:warning()
- ConsoleOutput:error()
The Console Output library is optional. In order to use this library, you must require it in one of your source files (preferably main.lua) prior to use. It only needs to be required once in a single file. It is recommended you do this at the top of your main.lua file, outside of any functions or other code, and you may do this by using this code:
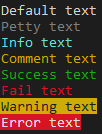
- default
- petty
- info
- comment
- success
- fail
- warning
- error
Instantiates a new Console Output object. The constructor has no side effects and accepts no parameters.
Configure a style for the console output object. The name
can be anything you would like to refer to this style as, and should be unique.
fmt
should be an ANSI-compatible format string. See ANSI console features for more information.
Returns whether ANSI console features are available on your machine.
This should, normally, return
Writes a raw string to the console. No newline will be appended for you.
Same as write(), except a newline will be appended for you.
Formats and returns a message (msg
) that is styled with the required style.
style
must be either the name of a default console style, or one that you have
created yourself with setStyle()
This function will not print the message to the screen; it will only prep the output for you and return it as a string.
Exactly the same as sstyle(), except it actually does print the message to the screen.
This will not append a newline for you, so be sure to include a "\n"
if needed.
Prints msg
to the screen using the 'default' style. This includes a newline.
This is a good choice for "normal" text.
Prints msg
to the screen using the 'petty' style. This includes a newline.
This is a good choice for insignificant text.
Prints msg
to the screen using the 'info' style. This includes a newline.
This is a good choice for informational messages.
Prints msg
to the screen using the 'comment' style. This includes a newline.
This is a good choice for code comments.
Prints msg
to the screen using the 'success' style. This includes a newline.
This is a good choice for success messages or other positive connotations.
Prints msg
to the screen using the 'fail' style. This includes a newline.
This is a good choice for when things go wrong, but are recoverable (ie. app will continue on).
Prints msg
to the screen using the 'warning' style. This includes a newline.
This is a good choice for warning messages.
Prints msg
to the screen using the 'error' style. This includes a newline.
This is useful for error messages, and indicates non-recoverable errors (ie. app will not be able to continue)
Page last updated at 2024-04-02 00:50:20