- Operators
- vector3d:set()
- vector3d:length()
- vector3d:normal()
- vector3d:dot()
- vector3d:cross()
- vector3d:rotateAboutX()
- vector3d:rotateAboutY()
- vector3d:rotateAboutZ()
- vector3d:rotateAbout()
- vector3d:lerp()
- vector3d:slerp()
- vector3d:moveTowards()
Operators for adding (+), subtracting (-), and scaling (* or /) are supported. You may also get the magnitude (length) of a vector with the # operator.
Change the x, y, and (optional) z axis for a
Returns the length (aka magnitude) of a vector. This is the same as the # operator.
Returns a normalized (vector with a magnitude of 1) copy of a vector.
Returns the dot product of a vector against some 'other' vector.
Returns the cross product of a vector against some 'other' vector.
Returns a copy of the vector rotated around the X axis by some angle in radians (not degrees!).
Returns a copy of the vector rotated around the Y axis by some angle in radians (not degrees!).
Returns a copy of the vector rotated around the Z axis by some angle in radians (not degrees!).
Returns a copy of the vector rotated around a user-specified axis by some angle in radians (not degrees!).
Linearly interpolate (lerp) a vector to rotate it towards the target vector by 'ratio' amount. 'ratio' should be between 0 (no rotation) and 1 (full rotation). Linear interpolation is quicker to calculate but less accurate than spherical linear interpolation (slerp).
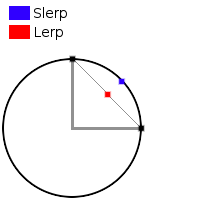
Lerp-ing will find a point that fits linearly between two other points in 3d space. On the other hand, if you were to visualize a slerp, it would track as if following the outside of a sphere. You might imagine a slerp being like the path an airplane would travel over the surface of the Earth, while lerp does not account for the curvature of the planet.
If this description doesn't seem very helpful, this video can explain the math and technical differences between the two, while this video provides a good visualization.
Spherical linearly interpolate (slerp) a vector to rotate it towards the target vector by 'ratio' amount. 'ratio' should be between 0 (no rotation) and 1 (full rotation). Spherical linear interpolation is slower to calculate but is visually smoother and more accurate than linear interpolation (lerp).
For a full description on the difference between lerp and slerp, see vector3d:lerp()
Move this vector up to 'dist' distance towards the target vector. This will automatically prevent overstepping the target should 'dist' be longer than the actual distance to the target. If distance is negative, this function will move away from the target vector.
Page last updated at 2018-09-25 20:48:56